A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company. Examples of working with text files. Writing and reading list, tuple, different types objects, dictionary, two dimensional matrix, set. In this tutorial, we will learn about python list append method. It adds a new element to the end of the list. Python Tutorial. Code section examples are given below: Using Markdown ```Python str = 'This is block level code' print(str) ``` Using Markup Tags Python str = 'This is a block level code' print(str).
This topic provides examples of writing and reading information for text files.
Contents
Search other websites:
1. Read/write list containing n integers
The following operations are shown in the example:
- creating a list of 10 random numbers;
- saving the list in a text file;
- reading from a file to a new list for control purposes.
Python Markdown Format
The text of the program is as follows:
The result of the program
⇑
2. Read/write list containing strings
When reading/writing lines, it is not necessary to implement additional conversions from one type to another, since the data from the file is read as lines.
The result of the program
⇑
3. Read/write tuple containing floating point objects
The example demonstrates writing and reading a tuple that contains floating point objects.
The result of the program
View of myfile5.txt file
⇑
4. Reading / writing a tuple containing objects of different types
If the tuple contains objects of different types during writing/reading, it is important follow to the sequence of stages of converting objects to the desired type. The following is an example of writing and reading a tuple that contains objects of integer, logical, and string types.
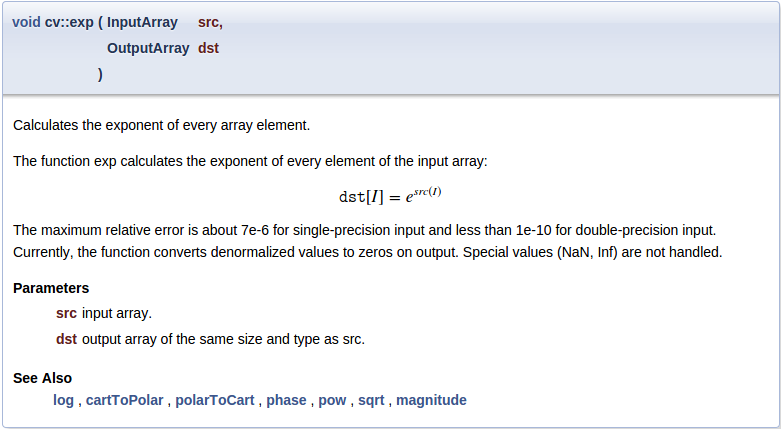
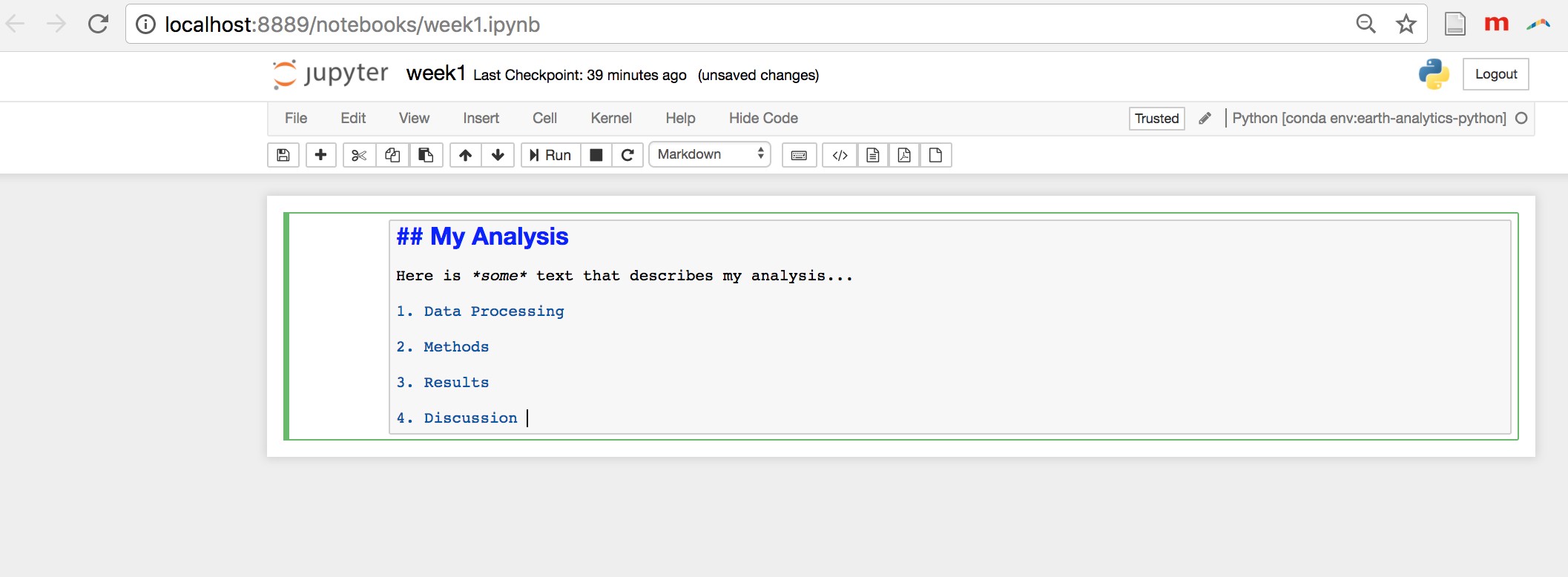
The result of the program
⇑
5. Read/Write Dictionary
The dictionary can also be written to a file. In this example, a dictionary is written and read that contains a list of weekday numbers and their names. To facilitate reading data, each element of the dictionary is placed on a separate string.
The result of the program
View of file myfile6.txt
⇑
6. Read/write two-dimensional matrix of integers, presented in the form of a list
The example demonstrates writing and reading a two-dimensional matrix of integers of dimension 3*4.
The result of the program
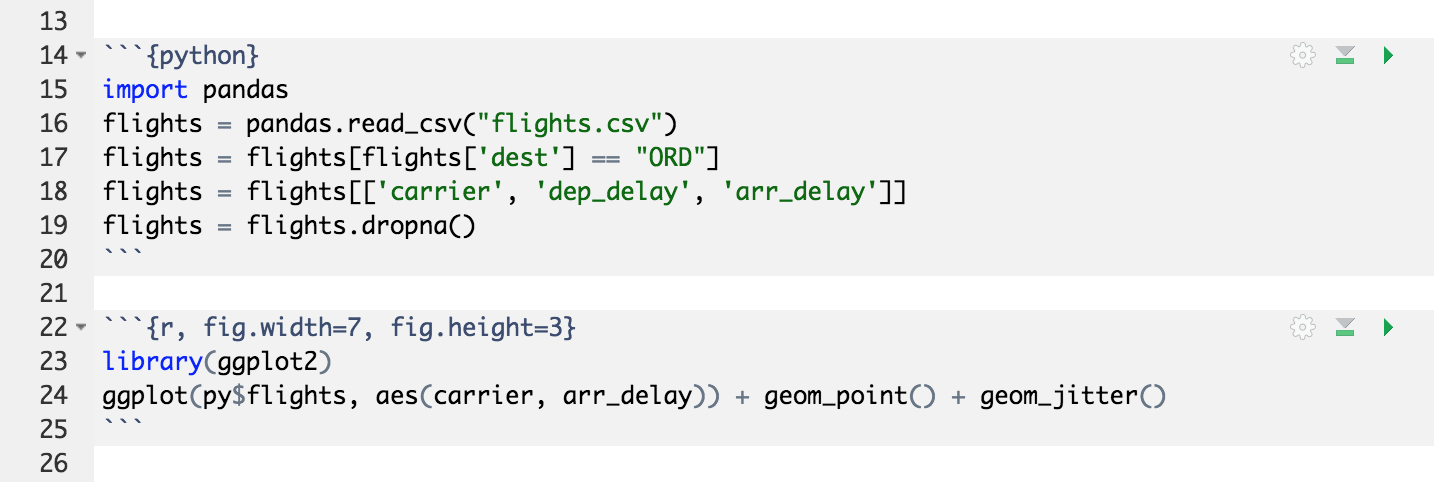
View of the file myfile8.txt
⇑
7. Read/write a set that contains integers
The example shows a possible way to save the set in a text file.
The result of the file
The view of myfile7.txt file
⇑
8. Read/write data of different types: list and tuple
To write data of different basic types to a text file, you need to sequentially write data of one type, then another type. When reading such data, one must adhere to this very order so as not to violate the resulting data structure.
The example demonstrates sequential writing to a list file and tuple. When reading, it follows the same sequence: first the list is read, then the tuple. The list includes strings. To facilitate the recognition of the file format, each written (readable) element is placed on a separate line in the file. Since the list and tuple may contain a different number of elements, their dimensions are written to the file.
The result of the program
The view of myfile9.txt file
⇑
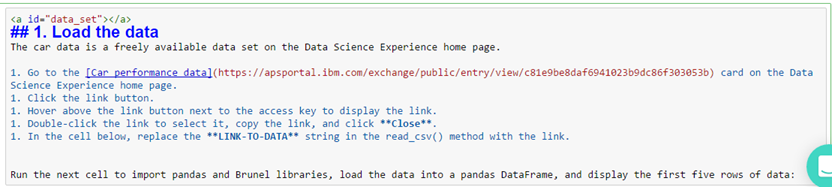
Related topics
Python Markdown Code
⇑
Python tutorial is a widely used programming language which helps beginners and professionals to understand the basics of Python programming easily.
Python is a high-level, easy, interpreted, general-purpose, and dynamic programming language. It supports object-oriented programming approach. It is straight forward to learn, and its elegant syntax allows programmers to express concepts in fewer lines of code as compared to other languages such as C, C++, or Java.
It has excellent>
Python 3.x:
2 | print('Hello World.') |
Usage of Python
Python is used by many programmers andused in various fields. Most of the time, it is used to perform any complicatedtask in a much easier way than other programming languages.
WhenPython is installed, it comes up with the many powerful libraries which made upof many functions.
Thereare many libraries available on the internet that makes it possible to docomplicated tasks more straightforwardly.
Thesestandard libraries make it a powerful programming language. Python is widely used in the followingfields:
- Web development,
- Software development,
- Machine Learning,
- GUIs based applications,
- Network programming,
- Game development,
- Mathematics
- Artificial Intelligence
- Data Science
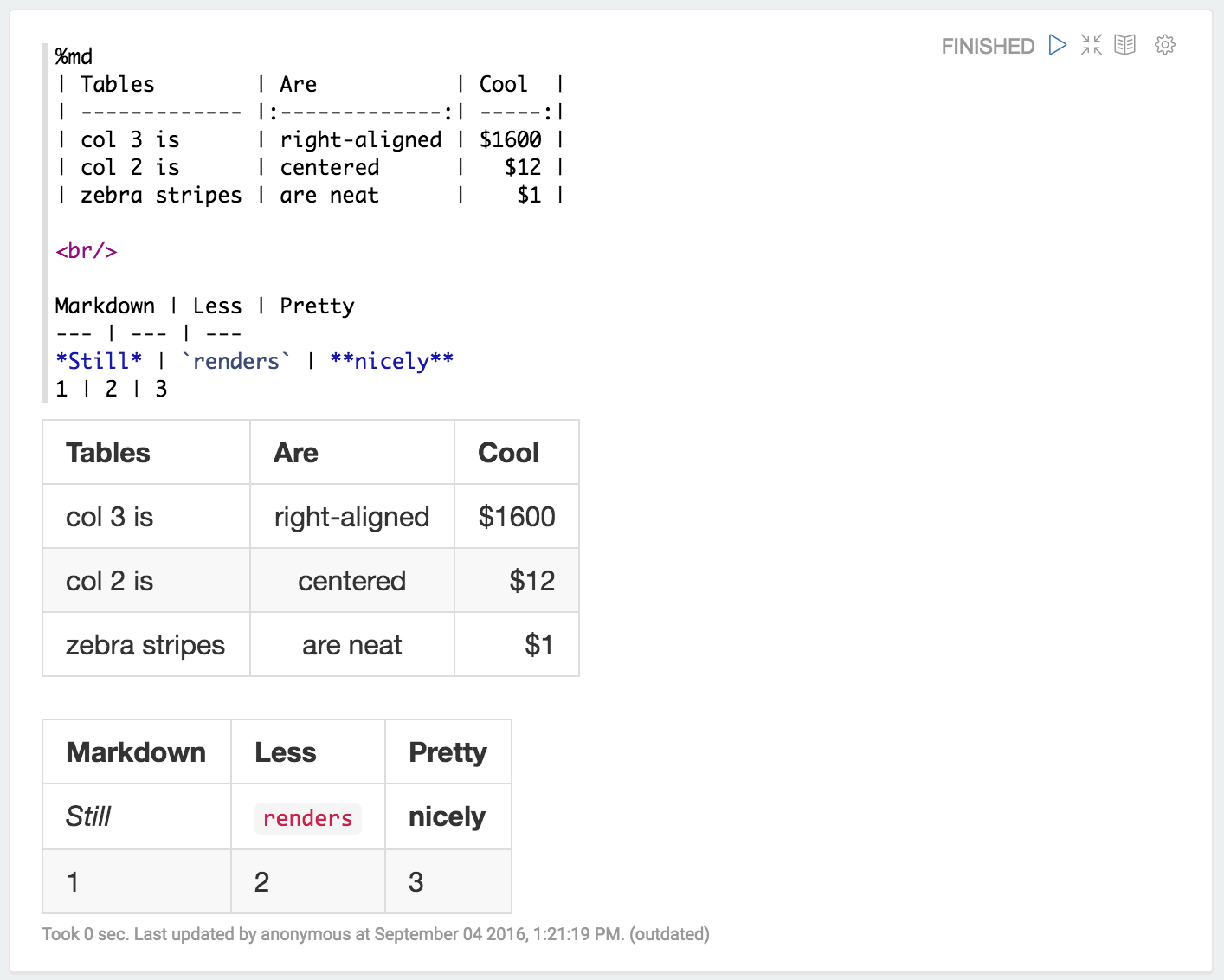
Why Python?
Here are some reasons why Python is the most popular language these days:
Pythonprovides rich libraries:
Python provides vast standard libraries that includeareas like machine learning,mathematics, web service tools, operating system interfaces, and protocols.
Python is Open Source language:
Python is freely available at its official site(www.python.org). Anyone can download Python from its official website
Easy to use:
Python is very easy to learn and easy to use. It is auser-friendly language. It uses a fewlines to express the code as compared to other languages.
For example- Suppose you want to print “Hello world”in java, code will be
2 4 6 8 | publicclassHelloWorld{ // Prints 'Hello, World' to the terminal window. } |
In Python, it canbe done by using one statement.
2 | print(“Hello World”) |
The output will bethe same in both codes, but in Python, it takes only one line.
Frameworks and Libraries
Python providesvarious frameworks for different development fields. There are some popular frameworksgiven below:
- Web development (Server-side): Django is the mostpopular web development framework of Python. Flask, Pyramid, CherryPy, andothers are also used for development.
- GUIs based applications: Tk, PyGTK, PyQt, PyJs, etc
- Machine Learning: TensorFlow, PyTorch, Scikit-learn, Matplotlib, etc
- Mathematics: Numpy, Pandas, etc.
Object-Oriented Approach
Python supportsobject-oriented programming approach. In object-oriented programming, OOPs concepts are based oncreating reusable code and solve programming problems by creating objects. Theseconcepts are also known as DRY(Don’t Repeat Yourself).
In Python, OOPsconcepts are given below:
- Classes
- Objects
- Inheritance
- Polymorphism
- Encapsulation
We will learnthese concepts in Python OOPs tutorial.
Extensible
Python isextensible, which allows invoking C and C++ library, and you can combine JAVAas well as .NET components.
Portability
Python is a portable language. For example, we have Python program for windows, and we want to run this code on other platforms such as Mac, Unix, Linux, then we do not need to change the code, we can run it on any platform. In this tutorial, we have covered a brief introduction to Python. Python is a very popular language and nothing wrong to say that it is the next generation language
Python Topics
- Python Example
- Python Basics
- Python Variables
- Python Break Statement
- Python Continue Statement
- Python Pass Statement
- Python Goto Statement
- Recursion in Python
- Python Iterator
- Lambda Function in Python
- Python OS Module
- Python Math Module
- Python Import Module
- Python Time Module
- Python Sys Module
- Python Requests Module
- Python Random Module
- Python Struct Module
- Python Calendar Module
- Python CSV Module
- Python Subprocess Module
- Python Namespace
- Exception Handling in Python
- Python Try Except
- Python Write CSV
- Python Read Text File
- Python Write Text File
- Python Read JSON File
- Python Write JSON File
- Python GUI Programming
- Python Network Programming
- Python Linked List
- Python Enumerate
- Classes and Objects in Python
- Inheritance in Python
- Polymorphism in Python
- Encapsulation in Python
- Python Super
- Python interface
- Python Logging
- Python Multithreading
- Python Compiler
- Python Interpreter
- Indentation in Python
- Speech Recognition in Python
- Face Recognition in Python
- Linear Regression in Python
- Logistic Regression in Python
- Python Rest API
- Python MySQL
- Python MongoDB
- Python Projects
- Python Exercises
Python Functions and Methods
Python Markdown Parser
Misc
- What is Python used for
- What is Anaconda Python
- Multiple Line Comment in Python
- Python Certification
- Python Counter
- Python Libraries
- Best Python Book
- Python Meaning | Python Definition
- Python Global Variable
- Python Open File
- Python Script
- Python Ternary Operator
- Python self
- Python vs Java
- Web Scraping Python
- Python Assert
- Python Def
- Top 10 Online Python Compilers / Editors
- Top 10 Python Frameworks
- Python Modulo
- Python Packages
- Python Syntax
- Python uses
- Python Bitwise Operators
- Python Command Line Arguments
- Python JSON
- Identifiers in Python
- Matrix Multiplication Python
- Python AND Operator
- Python OR Operator
- Python XOR Operator
- Python Logical Operators
- Python Multiprocessing
- Python New Line
- Python Subprocess
- Python Unittest
- Python Virutal Environment
- __init__ in Python
- Advantages of Python
- is Python case sensitive when dealing with identifiers
- NLTK Python
- Python Boolean
- Python Call Function
- Python History
- Python Image Processing
- Python KeyError
- Python Main Function
- Permutation and Combination in Python
- Difference between Input() and raw_input() functions in Python
- Sentiment Analysis Python
- Type Casting in Python
- Celery Python
- Collections in Python
- Conditional Statements in Python
- Confusion Matrix Python
- Decimal to Binary in Python
- Binary to Decimal in Python
- Escape Sequence in Python
- Literals in Python
- Nested For Loop Python
- Nested List Python
- Operator Overloading in Python
- Print Statement in Python
- Python Algorithms
- Python Attributes
- Python Commands
- Python Data Visualization
- Python Debugger
- Python DefaultDict
- Python Enum
- Python Glob
- Python Histogram
- Python Modules List
- Python Not Equal
- Python Null
- Python Raise Exception
- R vs Python
- Static Method in Python
- Static Variable in Python
- Who Developed Python
- Anaconda vs Python
- Difference between Python 2 and 3
- Heatmap Python
- Is Python Case Sensitive
- Method Overloading in Python
- Pointers in Python
- Python Arithmetic Operators
- Python Design Patterns
- Python Developer Salary in India
- Python File Operations
- Python Memory Management
- Python XML Parser
- Tic Tac Toe Python
- Assignment Operator in Python
- Indentation Error in Python
- Is Python Object Oriented
- Python yield
- Python return
- Python del
- Python Operator Precedence
- Python Project Ideas
- Python Deep Copy and Shallow Copy
- Python Division
- Python exit commands: exit(), quit(), sys.exit(), os._exit()
- Python HTTP Server
- Python **kwargs
- Python NameError
- Python Parser
- Module Not Found Error in Python
- SSL Module in Python is not available
- Difference between Package and Module in Python
How to
Python Format Markdown Table
- How to reverse a string in Python
- How to run Python Program
- How to take input in Python
- How to check Python version
- How to install Python in Windows
- How to install Python in Ubuntu
- How to install PIP in Python
- How to call a function in Python
- How to convert list to string in Python
- How to download Python
- How to check Python version in cmd
- How to convert string to int in Python
- How to convert int to string in Python
- How to define a function in Python
- How to install numpy in Python
- How to install pandas in Python
- How to comment multiple lines in Python
- How to create a file in Python
- How to create a list in Python
- How to create virtual environment in Python
- How to declare array in Python
- How to print in Python
- How to run python file in cmd
- How to clear screen in Python
- How to convert string to list in Python
- How to install OpenCV in Python
- How to make a game in Python
- How to print in same line in Python
- How to take multiple inputs in Python
- How to write a program in Python
- How to compare two strings in Python
- How to create a dictionary in Python
- How to create an array in Python
- How to declare variable in Python
- How to install matplotlib in Python
- How to update Python
- How to add elements in list in Python
- How to compare two lists in Python
- How to concatenate two strings in Python
- How to input a list in Python
- How to install CV2 in Python
- How to install library in Python
- How to install Tkinter in Python
- How to plot graph in Python
- How to remove element from list in Python
- How to reverse a number in Python
- How to sort a string in Python
- How to sort dictionary in Python
- How to split string in Python
- How to split a list in Python
- How to write a function in Python
- How to add Python to Path
- How to check data type in Python
- How to convert float to int in Python
- How to convert string to float in Python
- How to create a class in Python
- How to create a dataframe in Python
- How to declare global variable in Python
- How to find length of list in Python
- How to find length of string in Python
- How to find prime numbers in Python
- How to find square root in Python
- How to import numpy in Python
- How to import pandas in Python
- How to print pattern in Python
- How to round off in Python
- How to run python script in Linux
- How to uninstall Python
- How to upgrade PIP in Python
- How to add elements in dictionary in Python
- How to append string in Python
- How to connect MySQL with Python
- How to create object in Python
Searching
- Binary Search in Python
- Linear Search in Python
Sorting
- Python Sort Array
- Python Sort Dictionary
- Python Sort String
- sort() function in Python
- Bubble Sort Python
- Insertion Sort Python
- Merge Sort Python
- Selection Sort Python
- Quick Sort Python
- Sort Dictionary by Value in Python
- Sort Dictionary by Key in Python
- Sort and Sorted in Python
Programs
- Python Basic Programs
- Simple Python Program
- Pattern Program in Python
- Hello World Program in Python
- Number Pattern Programs in Python
- Python string programs
- Python array programs
